C# for Developers
- C#DL
Description
C# is an object oriented programming language developed by Microsoft that
enables developers to quickly build a wide range of applications for the
.NET platform. For the C# For Developers training course from ATS, some
programming experience will be helpful but none is assumed. Expert instructor
Jesse Liberty begins with a solid introduction to programming methods, shows
you how to build applications and continues with advanced topics like class
libraries, threading streams and interoperations with COM. At the conclusion
of this course, you will understand how to build Windows and Web applications
on .NET using C#.
|
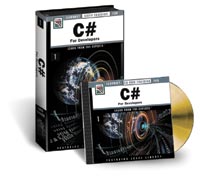 |
Course Outline
Module 1
Section A: C# & .NET Introduction The .NET PLATFORM
.NET Features .NET Process
Section B: C# Example Getting Started Set Text Set Up Button
View Event Handler Build & Run C# Basic Components
Classes, Objects, Types Methods
Section C: Console Apps Using Comments Console Object Name
Spaces Dot Operator Create Console App with Notepad Compile
& Run Visual Studio Console App Build & Run
Section D: Variable & Constants Declarations Replaceable Parameter
Variable Assignment Constants Set Variable Variable
Usage Set Enum Variable
Section E: Types, Expressions & White Space Types String
Identifiers Statements & Expressions White Space
Section F: Branching Basics Branch Types Unconditional
Basic Branching Return Statement Parameters & Local Variables
Debug Branch Conditional if
Section G: Switch & Iteration Statements Switch Expression
Convert String to Integer Use Switch Statement Iteration Types
For Statement Using Iteration Statements While & Do
While For Loop
Section H: Operators Usage Relational Mathematical
Logical Operators Short Circulation Evaluation Using Math Operators
Modules Operator Prefix & Post Fix Testing Logical
Module 2
Section A: Classes & Constructor Overview Access Modifiers
Methods of a Class This Keyword Default Constructor
Class Variables Using Class Add Member Variable Add Constructors
Review & Run
Section B: Static Methods & Destroying Objects Static Methods
Static Members Using Static Member Destroying Objects
Section C: Member Variables & Properties Types Stack &
Heap Passing Parameters Pass by Reference Return Multiple
Values Out Keyword Properties Using Get & Set
Section D: Inheritance Hierarchy Levels Derived Inheritance
Polymorphism Override Method Pass Type Declarations
Step Through
Section E: Boxing & Operator Overload System.Object Boxing
Operator Overloading Conversion Operators Creating Type-Roman
Compute Value Step-Through Overload Assign New Values
Implicit Conversion Explicit Conversion
Module 3
Section A: Interfaces Overview Define & Implement Interface
Implement Additional Classes Testing Implementation Create
Instance Create New Interface Apply Interface Define Extended
Interface Use Extended Interface Implement Extended Interfaces
Test Extended Interface Interface as Parameter Is Keyword
Step-Through Is Code As Keyword
Section B: Arrays Overview Foreach Loop Create Array
Use For & Foreach Initialize Array
Section C: Multi-Dimensional Arrays Dimension Types Rectangular
Array Initialize Array Dimensioning Issues Jagged Arrays
Params
Section D: Indexers Overview Create Indexer Create &
Fill Array Offset Into Array Stung Indexer
Section E: IEnumerable Overview Implement IEnumerate Using
IEnumerated Objects Step-Through Enumeration
Module 4
Section A: IComparable . Array Lists . IComparable Interface . Implement
IComparable . Custom Comparison . Custom IComparable . Runtime, Sort Choice
. Create Custom Compare
Section B: Collection Classes . Overview . Queues . Using Queue Class . Stacks
. Using Stack Class . Stacks to Arrays . Dictionary & Hashtable . Using Hashtable
Section C: Strings . Overview . Methods, Properties . String Builder Class .
Regular Expression . String Manipulations . String Properties & Methods . Splitting
within Strings . Splitting with Regular Expression
Section D: Exceptions . Overview . Try-Catch & Finally . Throw On Exception
. Using a Catch Block . Special Exception Handler . Finally Block . Using Help
& Stack Track
Section E: Nested Exceptions . Overview . Declare Custom Exception . Looping
Catch Block . Create Nesting . Step-Through Nesting
Section F: Delegates . Overview . Delegating Methods . Implementing Delegate
. Delegates as Parameters . Step-Through Delegated Function . Use Delegate to
Invoke Method . Static Delegates . Delegates as Properties
Module 5
Section A: Multicast Delegates . Overview . Using Multicast . Invoke Multiple
Methods
Section B: Events . Implementing Events . Sample Event Program . Event Handling
. Event Notification . Run Program . Register Delegate to Event . Create Event
Args Instance . Fire New Event
Section C: Windows Applications . Windows vs. Web Apps . Basic Window Form .
Hand Coding Windows App . Save, Compile . Use Visual Studio.Net . Modify Application
Section D: Windows Forms . Create New Project . Checkbox . Radio Buttons . Event
Button . Output Label . Checklist Box . Additional Controls . View Code & Run
Section E: XML Comments . Overview . Using XML Comments . Create XML Documentation
. Create HTML from XML Comments
Section F: Introduction to Relational Databases . Overview . Relational Databases
. Database Structure . SQL Server Diagrams . Structured Query Language . Query
Analyzer . Building Queries . Refining Information
Module 6
Section A: ADO.NET Objects Object Model Data Objects Create
ADO Application Using SqlDataAdapter Populate Listbox from Database
Using OleDbDataAdapter
Section B: Data Relationships Use DataGrid in ADO Application
Setup Connection Object Setup Command Object & Data Adapter
Populate DataGrid & Test Application Data Relationship Add
Additional Tables to DataGrid Data Relation Object Test &
Debug ADO Application Using Debug Tools Using ADO Application
Section C: Updates Overview Populate Listbox & Dropdown Menu
Update Displayed Data Update Dropdown Menu Update Database
Data Working in Multiple Tables
Section D: Transactions Overview ACID Test SQL Transactions
& Stored Procedures Use Stored Procedures Pass Parameters
to SPs Transaction Support with SP Connection Transaction
Commit & Rollback
Section E: Update Using Data Adapter Update DataSet Update DataSet
Method Map Column Names & Text Update Database with ADO.NET
Use DataAdapter to Update
Module 7
Section A: Concurrency Overview SPROC Params for Concurrency
ADO.NET with Concurrency Command Builder
Section B: Web Forms Overview Web Forms Diagram Web Forms
Support State Create ASP.NET Web App Add Label & Button
Add Button Run Web App Controls ASP.NET Events Create
DataGrid Bind DataGrid Customize DataGrid Controls Create
New Method Create DataRow View Extract DataItem Modify
DataCell by Event Handling
Section C: Attributes Overview Attribute Targets Using
Targets Using Attributes Create Attribute Named Attributes
ILDSM
Section D: Reflections Overview Create Array of Attributes
Use Discovery Process LateBinding
Section E: Threads Multithreading Support Time Splicing
Create Implicit Threads Joining Threads Killing Threads
Sleep Join Threads Abort Thread
Module 8
Section A: Synchronization Overview Concurrency Issues
Interlocked Increment Lock Threading Problems
Section B: Streaming Data Overview ExploreDirectory Read
& Write Streams Buffered Stream StreamReader & StreamWriter
Section C: Asynch I/O Overview AsyncCallback Delegate Test
Constructor Buffers and Tokens Buffering at Work HTTP Web
Request Web Streaming Objects Read Page from Web
Section D: Network I/O Network Streaming Sockets & Ports
Support Network I/O Server Side Client Side Server &
Client Interaction Asynch Network I/O Client Handler Asynch
Read & Write Send Text String Asynch File Read Asynch
File Streaming
Section E: Serialization Overview Binary Serialization
Serialize to Disk Deserialize Nonserialized Option
Section F: ActiveX Overview Create ActiveX Control Create
VB Project ActiveX Event Handler Import into .NET Add Control
to Toolbox Create .NET Project
Price £1250 (Bundle of 8)
Complementary Courses
VB to VB.net Migration
VB.net for Developers Part 1
VB.net for Developers Part 2
<<Back
<<Contact Us
|