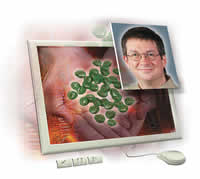 |
Java
2 Standard Edition - J2SE
Description
Java2 Standard Edition (J2SE) is Sun Microsystems software development
kit for building mission critical enterprise applications. In the ATS training
course J2SE for Developers, experienced programmers and project managers
responsible for creating enterprise applications using Java2 learn how to
use the tools within the SDK to provide rapid development and deployment.
Expert instructor Neal Ford starts with an introduction to Java2 and progresses
step-by-step to advanced topics. At the conclusion of this course, you will
understand how to design and implement an application using Java2. |
Benefits
Increase your earning potential and technical credibility.
Enhance employment opportunities with in-demand programming knowledge.
ATS courses let you move along at your own pace and gain new skills in
a useful, productive manner. .
Course Outline
Module 1
Section A: Introduction to Java · CD Tour · Java Basics ·
Types · Advantages · Platform Goals · Language Goals
· C++ Enhanced · Misconceptions
Section B: SDK Install & Setup · Java Acronyms · JRE/SDK
Relationship · SDK Install · Directory Structure
· Classpath · Set Path Options · Run Application ·
Run Applets
Section C: SDK Tools · javac Command · Test Compiler ·
java Command · javaw Command · jdb Command
· Run Apps · javadoc Command
Section D: IDEs · Open Source/Free · Commercial · Jext
· jEdit · Eclipse
Section E: Basic Syntax · Variable Basics · Data Types ·
Operators · Bitwise Operators · Unary Operators
· Other Operators · Conversions
Section F: Strings · Reference Types · String Class ·
StringBuffer · String Behavior · Compare Methods
· Server-Side Comparison · substring() Method
Section G: Flow Control · while Loop · do...while Loop ·
for Loop · Break/Continue · if...else Construct
· switch Construct · Ternary Operator
Module 2
Section A: Section A: OOP · Introduction to OOP · Java Classes
· main() Method · Garbage Collection
· toString() Method · raiseSalary() Method · Static Methods
Section B: More on OOP · Parameters/Designations
· Pass by Value · Constructor Overload · this Keyword ·
Tester Class · Employee Array
Section C: Encapsulation · Overloading · Private Scope ·
Accessors/Mutators · get & set · Validate Data
Section D: Packages · Name Error · Import · Classpath
Section E: Inheritance · extends Keyword · Manager Class ·
Parent Constructor · Override vs. Overload
· Child/Parent Relation · Test Manager Class
Section F: Polymorphism · Scope Within Packages · Scope Across
Packages · Polymorphism Example
· References/Arrays · instanceof Operator · Typecasting
& Object Class
Section G: Exception Handling · Runtime Errors · try/catch Block
· Unhandled Exceptions · Handled Exceptions
· Resource Protection · try Block Scoping · Rethrow Exceptions
Module 3
Section A: Runtime Exceptions · MyCustomException · Exception
Hierarchy · Checked/Unchecked · Use Java Source
· Catch IOException · Throws Clause · Empty Catch Blocks
Section B: JDBC · DB Connection/SQL Stmt · Driver Types ·
JDBC-ODBC Bridge · Native-API Driver
· Net-Protocol Driver · Native-Protocol Driver · Driver
Selection · Connection Process
Section C: Using JDBC · Download Driver · Critical Data ·
Create Constants · Access/Load Driver Class
· Use DriverManager · JDBC Classes · Exception Handling
· JDBC Driver JAR File Section D: MetaData
· Setup Driver · getConnection · ResultSetMetaData ·
Iterate Through Columns · Test & Run
Section E: Simple Deployment · Runtime Environment · Classpath
Environment · Apps with Packages · Graphic Application
· External Dependencies
Section F: JAR Files · Java Archives · JAR Options · Create
JAR File · Contents & Update · Self-Executing JARs
· Manifest Entry · Executable JAR File · External Dependencies
Section G: Advanced Java Syntax · Using StringBuffer · Allocating
StringBuffers · String Methods · Graphic Application
· StringTokenizer · Arrays · Constant Arrays · Array
Operations
Section H: Abstract Classes · Final Modifier · Garbage Collector
· Characteristics · Sortable Abstract Classes
· compareTo() Method · Implementation · Spanning Hierarchies
Module 4
Section A: Interfaces · compareTo() Method · Arrays.sort() Method
· Comparator Interface · Apply to Sorting
· Tagging Interfaces
Section B: Inner / Anonymous Classes · Inner Class · Compiled
Files · Anonymous Class · ExtraClassFrame
· InnerClassFrame · Compiler View
Section C: Cloning · Shallow Copying · Deep Copyin · Cloneable
Interface · Protected Clone · Cloning Primitives
· Inheritance · Calling Clones · Cloning Strings
Section D: Advanced OOP Methods · equals() Method · Symmetric
Principle · Transitive Principle · With Composition
· Other Principles · Equals Checklist · hashCode() Method
Section E: Intro to Threading · run() Method · Thread Types ·
interrupt() Method · Priorities · Inheriting from Thread
· Runnable Interface · Running Multiple
Section F: Thread Synchronization · Synchronized Block · Setup
Threads · IllegalMonitorState · Using Synchronized
· notifyAll() Method · Deadlocks
Section G: Frame Basic · Frame Hierarchy · Ancestors ·
BasicFrame · Close Operations · Create a Frame · Center
a Frame
· Containership
Module 5
Section A: Layout Managers · JBuilder · Containership Hierarchy
· Layout Manager Basics · BorderLayout Basics
· BorderLayout Controls · FlowLayout Basics · FlowLayout
Controls
Section B: Intermediate Layout Manager · GridLayout Basics ·
GridLayout Controls · GridLayout Sizing
· BoxLayout Basics · Controls with Code · Strut/Glue Spacers
Section C: Advanced Layout Manager · GridBagLayout Basics · GridBagConstraints
· Null to GridBag
· Control Constraint View · Source Code · Disadvantages
· View Layout Design
Section D: Multi-Window Application · Modal Dialog · Frame/Dialog
Disposal · InputNameDialog · Dialog Event
· JDialog vs. Child Frame Section E: Swing Controls · History
· AWT vs. Swing · Swing Look & Feel · JLabel
· JTextField/JTextArea · JScrollBar · JButton ·
JProgressBar
Section F: More Swing Controls · JSlider · JRadioButton/Button
Group · JPanel · JScrollPane · JTabbedPane/JSplitPane
· JOptionPane · Message/Option Types · SwingSet Controls
Module 6
Section A: Event Handling · Generate/Dispatch · ButtonHandler
· ActionListener · WindowListener · WindowAdapter
· Internal/External · Access to Controls · Anonymous/Inner
Class
Section B: Model-View-Controller · MVC & Swing · JList ·
ListModel · Update ListModel · Default ListModel
· TableModel · Create TableModel
Section C: Advanced JDBC · JDBC & MetaData · ResultSet ·
Column Count · The Frame · Row Count · Demo
· Prepared Statement · Handling Keys
Section D: Files Class · Streams · Hierarchies · How Streams
Work · Layer Streams · Construct Frame
· JFileChooser · File Properties · List Files
Section E: Streams & Readers · Properties File · Write Properties
· Read Properties · Generate Exceptions
· PrintWriter · View Exception Log · Read Exception Log
· Log Info Objects
Section F: Helper Class · Type Wrapper Class · Using Wrapper Class
· Collections API · Using Vector Elements
· Create Enumeration · HashTable · Other Collection Classes
· Declarations
Module 7
Section A: Applying Collections · Convert Array Elements · Modify
Helper Method · Modify Print Loop · Collections Option
Section B: Calendars · Date Classes · Math Classes · Calendar
Class · Calendar Constants · Using Calendar Class
· CalendarTableModel · Use CalendarJTable
Section C: Formatting · NumberFormat · Custom Mask · Internationalization
· Break at Dots
Section D: Applets · Issues · Java Plug-in · Methods ·
Security Restrictions · Applets in HTML · Deploying Applets
· Applet Building / Tools
Section E: Applet Implementation · AWT Applet Basics · HTML Codebase
· Applet Viewer · AWT Applet in Browser
· SwingApplet · Plug-in Control Panel · SwingApplet in
Browsers
Section F: JavaBeans · Characteristics · Requirements ·
Application Builder · Building JavaBeans · Uses
· Create JavaBeans · Use I Component · Update JavaBean
Section G: Non-Visible JavaBean · Encapsulated Module · Get &
Set Methods · Convert Class
Section H: JTrees · Complex Swing Control · Build Tree Model
· Return Child index · Instantiate Tree Model
Module 8
Section A: Web Foundations · CGI · ISAPI / NSAPI ·
Servlets · Servlet Engines · Servlet Details
Section B: Servlet Foundations · Tomcat Servlet Engine · Build
Servlet Object · Servlet Methods · doGet / doPost
· Install & Run · Friendly Name · Servlet Using Parameters
· Pass Parameter Values
Section C: Servlet Parameters · Passing Parameters · Get vs.
Post · Use HTML Form Tag · Init Parameters
· Context Parameters
Section D: Servlet Threading · Threading Issues · Servlet Thread
Protection · Synchronized Blocks
Section E: Servlet Session Tracking · Stateless Solutions · HttpSession
Object · Persist Web Page Info
· Get Data From Session
Section F: JSP Foundations · JSP Benefits · JSP Documents ·
Generated Servlet
Section G: Elements of JSP · Markup Tags · Directives Attributes
· Scripting Elements · Comment Types
· Bean Tags · Using Beans in JSP · Naming Convention ·
Set Property
Section H: Java Web Start · Web Start Client · JNLP ·
Functionality · Application Manager · Launch App
· JNLP File · Security Model
Price £1195 (Bundle of 8)
Complementary Courses
AJV1 - AS/400 Java Introduction (mainly for AS/400 Developers)
JAV2 - Java 2 for Developers
JA2E - Java 2 Enterprise Edition
XMJV - XML in Java
JAS1 - JavaScript for Developers Part 1
JAS2 - JavaScript for Developers Part 2
<<Back <<Contact
Us
|